Here’s a quick video of this power feed in action. This is a simplified version without a display, it just does direction and speed. Feed rate can be adjusted at any time independent of the spindle RPM.
Here is a circuit diagram for this version:
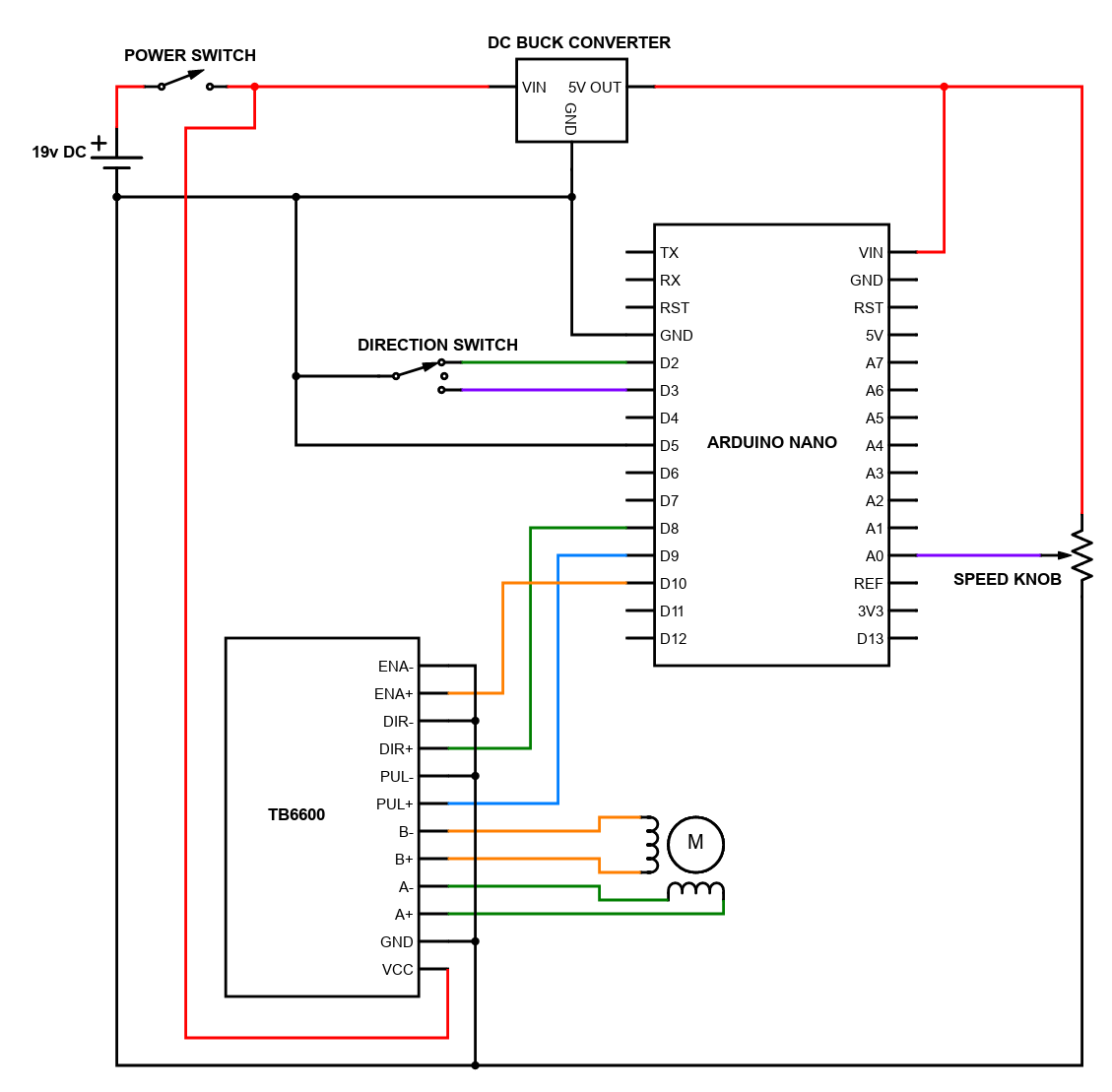
And here is the code running on the arduino:
//Mini lathe power feed. https://elliotmade.com/2020/08/03/mini-lathe-power-feed-demo/
//need validation for the right/left positions and current position
//////////////////////////////////////////////libraries////////////////////////////////////////////////////////////
#include <SimpleTimer.h>
//https://github.com/jfturcot/SimpleTimer
#include <AccelStepper.h>
//http://www.airspayce.com/mikem/arduino/AccelStepper/
#include <Ewma.h>;
//https://github.com/jonnieZG/EWMA
//////////////////////////////////////////Pins////////////////////////////////////////////////////////////////
const int leftPin = 2;
const int rightPin = 3;
const int speedPin = A0;
const int stepPin = 9;
const int dirPin = 8;
const int enablePin = 10;
////////////////////////////////////////////Configuration//////////////////////////////////////////////////////////////
const int speedMult = 2; //multiplier used for max steps/sec. curSpeed (0-100) * speedMult = steps per second
const int maxAccel = 1000; //steps/sec squared
//operation modes
const byte left = 0;
const byte right = 1;
const byte neutral = 2;
byte curDirection = neutral; //the first time this is checked it will always be different than 3
////////////////////////////////////////////Variables//////////////////////////////////////////////////////////////
int curSpeed = 0;
//Initialize some things
AccelStepper motor(1, stepPin, dirPin);
SimpleTimer timer;
Ewma filteredSpeed(0.1);
void setup() { //Setup
digitalWrite(enablePin,HIGH);
//configure the stepper library
motor.setMaxSpeed(4000);
motor.setAcceleration(maxAccel);
//pin configuration
pinMode(leftPin, INPUT_PULLUP);
pinMode(rightPin, INPUT_PULLUP);
pinMode(enablePin, OUTPUT);
}
void loop() { //Loop
readDirection();
readSpeed();
motor.runSpeed();
}
void readSpeed() { //update the speed knob reading except for auto mode
curSpeed = filteredSpeed.filter(analogRead(speedPin)* speedMult);
if (curDirection == neutral) {
motor.setSpeed(0);
}
else {
if(curDirection == left) {
motor.setSpeed(curSpeed);
}
else {
motor.setSpeed(-curSpeed);
}
}
}
void readDirection() {
if(digitalRead(leftPin) == LOW) {
curDirection = left;
}
else if(digitalRead(rightPin) == LOW) {
curDirection = right;
}
else {
curDirection = neutral;
}
}
If you’d like to build this for yourself, more details can be found on this post. It is powered by a 19v laptop charger, an arduino, and a tb6600 stepper driver.